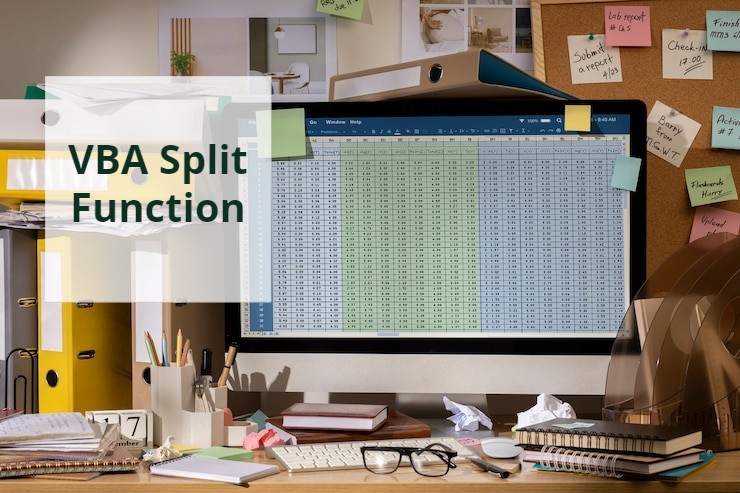
VBA Split Function: A Guide for Excel Automation
Introduction to the VBA Split Function
Welcome to an insightful journey into the world of Excel VBA programming, specifically focusing on the powerful and versatile VBA Split function. This function is a hidden gem in the realm of text manipulation, an essential skill for any VBA programmer.
Text manipulation is an integral part of VBA programming, largely due to the data-centric nature of Excel. Whether it’s preparing data for analysis, parsing information from large text files, or automating report generation, the ability to skillfully manipulate text data is crucial. The Split function, with its simplicity and efficiency, plays a pivotal role in these tasks, making it an indispensable tool in the VBA toolkit.
At its core, the VBA Split function is designed to dissect a string of text into an array, with segmentation determined by a specified delimiter, such as a comma, space, or colon. This functionality is not just a convenience; it’s a cornerstone in efficient data manipulation and analysis within Excel. It allows programmers to easily break down complex strings into manageable and analyzable parts.
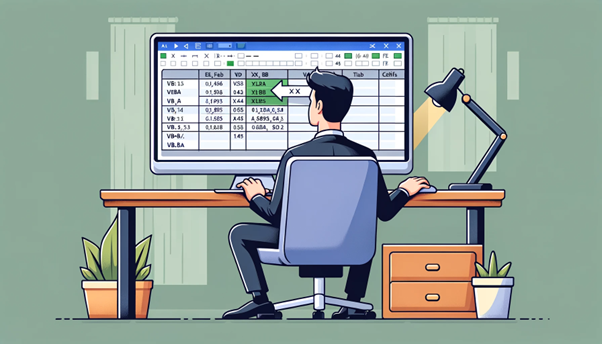
VBA Split Function – Syntax and Arguments
The basic syntax of the Split function is expressed as:
Split(expression, [delimiter, [limit, [compare]]])
It returns a zero-based, one-dimensional array containing a specified number of substrings.
Argument | Optional / Required | Description | Note |
expression | Required | The string to split | Returns an empty array if the expression is a zero-length string (“”). |
delimiter | Optional If omitted, a space character (” “) is assumed. |
The character to delimit the boundaries of substrings. | If the delimiter is a zero-length string, the entire expression string is returned as a single-element array. |
limit | Optional The default value is -1, which means all substrings are returned. |
Indicates the number of substrings to return. | Â |
compare | Optional | Dictates the type of comparison, i.e. binary or textual. | vbBinaryCompare (0) for binary (case-sensitive) comparison, or vbTextCompare (1) for textual (case-insensitive) comparison. |
VBA Split Function – Basic Usage Examples
Here, we’ll present some simple examples to demonstrate its usage, how to split a text string into an array, and how to access individual elements of the resultant array.
Splitting a Sentence into Words Using the Default Space Delimiter
Sub SplitWords()
Dim textString As String
Dim result() As String
textString = "The Quick Brown Fox Jumps Over The Lazy Dog"
result = split(textString)
MsgBox Join(result, vbCrLf)
End Sub
Using a Custom Delimiter
Sub CommaSeparator()
Dim textString As String
Dim result() As String
textString = "Apple,Orange,Banana"
result = split(textString, ",")
MsgBox Join(result, vbCrLf)
End Sub
Utilizing the Split to Count Words
Sub WordCount()
Dim textString As String
Dim result() As String
TextString = "The Quick Brown Fox"
result = Split(textString)
MsgBox "The Word Count is " & UBound(result) + 1
End Sub
Accessing Individual Elements of the Resulting Array
Once a string is split into an array, accessing individual elements is straightforward. Each element in the array can be accessed using its index, starting with 0 for the first element. For example:
Sub AccessElements()
Dim result() As String
result = split("One,Two,Three", ",")
secondElement = result(1)
MsgBox "Second Element: " & secondElement
End Sub
Handling Consecutive Delimiters
In situations where multiple delimiters appear consecutively, it’s important to decide whether to treat them as one or separate them.
Sub HandleConsecutiveDelimiters()
Dim dataString As String
dataString = "apple,,orange,,banana,,grape"
Dim fruits() As String
fruits = split(dataString, ",,")
MsgBox Join(fruits, vbCrLf)
End Sub
In this example, the consecutive commas are treated as a single delimiter. If each comma was treated individually, it would result in empty strings in the array where the commas are consecutive.
Empower your business with our Excel Programming and VBA Macro Development Services, tailored to automate tasks and unlock the full potential of your data management capabilities.
Working with Limit and Compare Arguments in the VBA Split Function
As mentioned in an earlier section, the VBA Split function includes two additional arguments, Limit and Compare, which offer enhanced control over how strings are split and compared. Understanding these arguments is crucial for fine-tuning the function to meet specific needs.
Limit Argument
- Purpose: The ‘Limit’ parameter is used to define how many splits should be performed on the string.
- Default Behavior: If ‘Limit’ is not specified or set to -1, the Split function will return all possible substrings.
- Usage: When you set a numerical value for ‘Limit’, the Split function restricts the number of substrings to that number.
Sub LimitSplit()
Dim textString As String
Dim result() As String
textString = "One,Two,Three,Four"
result = split(textString, ",", 2)
MsgBox Join(result, vbCrLf)
End Sub
In this example, the Limit is set to 2, so the function returns the first two substrings split by commas.
Compare Argument
The Compare argument determines the type of comparison used for evaluating substrings in the splitting process, especially relevant when the delimiter is a letter or a set of letters.
- Purpose: ‘Compare’ specifies whether the comparison is case-sensitive or case-insensitive.
- Options:
- ‘vbBinaryCompare’ (value 0): Case-sensitive comparison.
- ‘vbTextCompare’ (value 1): Case-insensitive comparison.
Sub UseCompare()
Dim textString As String
Dim result() As String
textString = "An apple with an oak"
result = split(textString, "an", -1, vbTextCompare)
MsgBox Join(result, vbCrLf)
End Sub
In this example, the UseCompare function splits the string at every occurrence of “an”, regardless of its case, due to the vbTextCompare option.
Combining Limit and Compare
Both Limit and Compare can be used together for more complex scenarios.
Sub CombineLimitAndCompare()
Dim textString As String
Dim result() As String
textString = "Apple And Orange and Banana"
result = split(textString, "and", 2, vbTextCompare)
MsgBox Join(result, vbCrLf)
End Sub
In this case, the string is split at the first two occurrences of “and” (case-insensitive), and the rest of the string is left intact as the second element of the array.
Combining Split with Other VBA Functions
The VBA Split function can be effectively combined with other VBA functions like Join, Trim, or Replace to enhance its utility in various scenarios. These combinations allow for sophisticated manipulation and restructuring of string data. Let’s explore some real-world examples to illustrate these techniques.
Combining Split with Replace
For more complex situations, such as different types of consecutive delimiters, the Replace function can be used in conjunction with Split.
Sub AdvancedDelimiterHandling()
Dim complexString As String
complexString = "word1,,word2;word3;;word4"
' Replace consecutive delimiters with a single character
complexString = Replace(complexString, ",,", ";")
' Replace consecutive delimiters with a single character
complexString = Replace(complexString, ";;", ";")
Dim result() As String
result = split(complexString, ";")
MsgBox Join(result, vbCrLf)
End Sub
Here, the ‘Replace’ function is first used to standardize the delimiters, replacing consecutive commas with a semicolon, which is then used as the delimiter in the ‘Split’ function.
Combining Split and Join
Scenario: We have a string of items separated by commas and want to transform it into a semicolon-separated list.
Sub CombineSplitAndJoin()
Dim originalString As String
Dim splitArray() As String
Dim newString As String
originalString = "Apple,Orange,Banana,Grape"
' Split the string into an array
splitArray = split(originalString, ",")
' Join the array elements into a new string with semicolons
newString = Join(splitArray, ";")
MsgBox newString
End Sub
This example uses Split to divide the string into an array and then Join to concatenate the array elements into a new string, separated by semicolons.
Combining Split and Trim
Scenario: Split a string into words and remove any leading/trailing spaces from each word.
After splitting the sentence into words, Trim is used in a loop to remove extra spaces from each word.
Sub CombineSplitAndTrim()
Dim sentence As String
Dim words() As String
Dim i As Integer
sentence = " Hello  World This Is VBA "
words = Split(sentence)
For i = LBound(words) To UBound(words)
words(i) = Trim(words(i))
Next i
MsgBox Join(words, vbCrLf)
End Sub
Advanced Techniques for Using the VBA Split Function
The VBA Split function can be leveraged for advanced text manipulation tasks, especially in parsing structured data and dealing with nested delimiters or complex text structures. Let’s delve into some sophisticated techniques and tips for efficient text parsing in VBA.
Parsing Structured Data
Scenario: We have a structured data string where each record is separated by a semicolon, and within each record, data fields are separated by commas.
Sub ParseStructuredData()
Dim DataString As String
Dim Records() As String
Dim Fields() As String
Dim i As Integer
DataString = "John,Doe,30;Jane,Smith,25;Mike,Brown,40"
' Split the string into records
Records = Split(DataString, ";")
For i = LBound(Records) To UBound(Records)
' Split each record into fields
Fields = Split(Records(i), ",")
MsgBox Join(Fields, vbCrLf)
Next i
End Sub
This method splits the data first by records and then by fields within each record, making it easy to process structured data strings.
Handling Nested Delimiters
Working with data where a primary delimiter encloses secondary delimiters.
Sub HandleNestedDelimiters()
Dim ComplexString As String
Dim PrimaryParts() As String
Dim SecondaryParts() As String
Dim i As Integer
ComplexString = "Name:John Doe;Age:30|Name:Jane Smith;Age:25"
' Split by primary delimiter "|"
PrimaryParts = Split(ComplexString, "|")
For i = LBound(PrimaryParts) To UBound(PrimaryParts)
' Further split by secondary delimiter ";"
SecondaryParts = Split(PrimaryParts(i), ";")
MsgBox Join(SecondaryParts, vbCrLf)
Next i
End Sub
In this example, the string is first split into larger sections using the primary delimiter, and then each section is further split using the secondary delimiter.
Â
Common Pitfalls and Troubleshooting in Using the VBA Split Function
While the VBA Split function is incredibly useful, users can encounter certain common pitfalls. Understanding these and knowing how to troubleshoot them is key to effective string manipulation. Let’s explore some of these issues and their solutions.
Incorrect Delimiter Usage
- Issue: Using the wrong delimiter or overlooking the case sensitivity of the delimiter can lead to unexpected results.
- Solution: Verify the delimiter and use the Compare argument for case sensitivity. Test the Split function with known input to ensure correct delimiter usage.
Handling Empty Strings
- Issue: If a delimiter is found at the beginning or end of the string, or if there are consecutive delimiters, the Split function might return empty strings in the array.
- Solution: Use the Trim function to remove unwanted spaces and check for empty strings in the array after splitting.
Ignoring the Zero-based Array
- Issue: Forgetting that the resulting array from Split is zero-based can lead to ‘Out of Range’ errors.
- Solution: Always remember that array indexing starts at 0. Use LBound and UBound to determine the array bounds.
Not Handling Variable-Length Arrays
- Issue: Assuming a fixed size for the result array can cause errors if the actual number of substrings varies.
- Solution: Dynamically manage array sizes using UBound to get the upper limit of the array.
Troubleshooting Tips
- Debugging the Code:
- Use the VBA debugger to step through the code. Check the values of variables before and after the Split function call.
- Validating Inputs:
- Ensure that the input string and delimiter are valid and as expected. Watch out for hidden characters or leading/trailing spaces.
- Array Bounds Check:
- Before accessing array elements, check if the index is within the bounds of the array to avoid ‘Subscript out of Range’ errors.
- Testing Edge Cases:
- Test with strings that include no delimiters, strings that start/end with delimiters, and strings with consecutive delimiters.
- Use of Immediate Window:
- Utilize the Immediate Window in the VBA editor to print out results and array contents for quick checks.
- Handling Null or Empty Inputs:
- Add checks to handle cases where the input string might be null or empty.
- Error Handling Implementation:
- Implement error handling using Try…Catch or On Error Resume Next to manage unexpected errors gracefully.
Best Practices and Efficiency Tips for Text Manipulation in VBA
Efficient text manipulation in VBA, especially when using functions like Split, requires adherence to certain best practices and optimization strategies. Let’s explore key approaches to ensure your code is efficient and readable.
- Use String Functions Judiciously
Minimize using string functions in loops, as they can be resource-intensive. Where possible, process strings outside of loops.
- Prefer Built-in Functions Over Custom Loops
Utilize built-in string functions (like Split, Len, InStr, Replace) instead of custom loops for string operations to enhance performance.
- Optimize Large Data Handling
When dealing with large texts (like processing data in Excel sheets), consider reading the data into an array first and then manipulating it. Interacting directly with sheet cells is slower.
- Batch Processing
For operations that need to be performed on multiple strings, consider batch processing to minimize the overhead of repeated function calls.
- Minimize String Operations: String operations can be resource-intensive. Try to minimize the number of operations by planning your parsing logic efficiently.
- Optimize with Regular Expressions: Consider using regular expressions via the VBScript for highly complex parsing tasks.RegExp object.
- Error Handling: Implement robust error handling, especially when dealing with unpredictable data sources.
- Use Early Binding: If you are using additional objects like RegExp, use early binding by setting a reference to the required library (via Tools > References in the VBA editor) for better performance.
Â
Optimizing Code with the Split Function
- Efficient Delimiter Handling
- Choose the most appropriate delimiter for the task. If the delimiter is not a single character, consider using the Replace function to simplify delimiters before splitting.
- Managing Resultant Arrays
- Always check the size of the array returned by Split using UBound to avoid ‘Out of Range’ errors and to loop through the array efficiently.
- Avoiding Unnecessary Splits
- If only a certain part of the string is needed, avoid splitting the entire string. Use functions like InStr and Mid to extract the required segment.
- Combining Split with Other Functions
- When more complex parsing is required, combine Split with other functions like ‘Trim’, ‘Join’, or Replace for more effective manipulation.
Enhance your software capabilities with our customizable Add-In Solutions, seamlessly integrating new features to meet your business needs.
Conclusion
In this comprehensive exploration of the VBA Split function, we’ve delved into its versatility and power as a tool for text manipulation within Excel VBA programming.Â
The true power of the Split function lies in its application. I encourage you, the readers, to experiment with this function in your VBA projects. Whether it’s data cleaning, preparation, or complex text parsing, the Split function can be an invaluable tool in your programming arsenal. Try combining it with other VBA functionalities to discover more efficient and creative solutions to your text manipulation needs.
Your experiences, questions, and feedback are vital to fostering a collaborative learning environment. If you’ve tried these techniques in your projects, have questions about specific scenarios, or have insights from your experiences, please feel free to share them. Your input not only benefits your learning journey but also aids fellow readers in enhancing their understanding and application of the VBA Split function.
Our experts will be glad to help you, If this article didn't answer your questions.
We believe this content can enhance our services. Yet, it's awaiting comprehensive review. Your suggestions for improvement are invaluable. Kindly report any issue or suggestion using the "Report an issue" button below. We value your input.